- What is object-oriented programming (OOP)?
- Object-oriented programming is a programming paradigm that is based on the concept of “objects”, which can contain data and code that manipulates the data. It is designed to be reusable and modular, making it easier to create and maintain complex software systems.
- What is a static method in OOP?
- A static method is a method that is associated with a class, rather than with a specific object. It can be called on the class itself, rather than on an instance of the class.
- What is a static field in OOP?
- A static field is a field (a variable) that is associated with a class, rather than with a specific object. It can be accessed on the class itself, rather than on an instance of the class.
- What is the difference between a class and an object?
- A class is a blueprint or template that defines the properties and behaviors of a particular type of object. An object is a concrete instance of a class, created at runtime.
- What is the difference between inheritance and composition in OOP?
- Inheritance is a way of creating a new class that is a modified version of an existing class. Composition is a way of creating a new class by combining the functionality of other classes. Inheritance involves a subclass inheriting the properties and behaviors of a superclass, while composition involves a class using the functionality of other classes through object references.
- What is the difference between an abstract class and an interface in OOP?
- An abstract class is a class that cannot be instantiated on its own but is intended to be used as a base class for one or more subclasses. It can have both abstract methods (methods with no implementation) and concrete methods (methods with an implementation). An interface is a set of related methods that a class can implement. It only contains abstract methods, and cannot have any concrete methods.
- What is the difference between method overloading and method overriding in OOP?
- Method overloading is the process of creating multiple methods with the same name, but with different parameter lists. Method overriding is the process of creating a method in a subclass that has the same name, return type, and parameter list as a method in the superclass, and providing a new implementation for the method in the subclass.
- What is the difference between a static and a non-static member in OOP?
- A static member is a member of a class that is associated with the class itself, rather than with a specific object. A non-static member is a member of a class that is associated with a specific object.
- What is a toString() method in OOP?
- The toString() method is a method that is defined in the Object class (the parent class of all objects in Java) and is used to convert an object to a string representation. It is often overridden in subclasses to provide a more meaningful string representation of the object.
- What is the difference between a field and a property in OOP?
- A field is a variable that is associated with a class or object. A property is a member of a class that provides access to a field but can have additional behavior associated with it, such as validation or notification when the value of the field changes.
- What is the difference between a static method and an instance method?
- A static method is a method that is associated with a class, rather than with a specific object. It can be called on the class itself, rather than on an instance of the class. An instance method, on the other hand, is a method that is associated with a specific object and can only be called on an instance of the class.
- What is the difference between polymorphism and overloading in OOP?
- Polymorphism is the ability of an object to take on multiple forms. It allows an object to be treated as a member of a superclass while still retaining its specific characteristics as a member of a subclass. Overloading is the ability to use the same method name with different sets of parameters in a single class. It allows a class to have multiple methods with the same name, but with a different number or types of arguments.
- What is the difference between encapsulation and data hiding in OOP?
- Encapsulation is the process of bundling data and methods that operate on that data within a single unit, or object. It is a way of protecting the data from outside access or manipulation and is an important concept in object-oriented programming. Data hiding is a technique used to protect the internal data of an object from being directly accessed or modified by external code. It is a way of implementing encapsulation in object-oriented programming.
- Can you explain the difference between a class and a struct in OOP languages? When should each be used?
- In object-oriented programming (OOP), a class is a blueprint for creating objects, while a struct is a lightweight composite data type that allows you to group together values with different data types.
- A class is used to define a more complex data structure, while a struct is used to define a simple data structure. A class can have attributes (variables) and methods (functions), while a struct can only have attributes. A class is generally used to model more complex real-world objects, while a struct is used to group together simple data values that are not complex enough to warrant the overhead of using a class.
- Here are some general guidelines for when to use a class or struct:
- Use a struct when you want to represent a small, simple data structure that contains a few basic data types. Structs are usually used to group together values that are all of the same type, such as a point in 2D space (with x and y coordinates).
- Use a class when you want to represent more complex data structures that may have a number of attributes and behavior (methods). Classes are often used to model real-world objects, such as a person, a bank account, or a computer.
- It’s worth noting that the differences between classes and structs can vary depending on the programming language you’re using. In some languages, classes and structs may have more or less functionality than in others.
- How do you implement the template method pattern in OOP? What is the purpose of using the template method pattern?
- The template method pattern is a behavioral design pattern that defines the skeleton of an algorithm in an operation, deferring some steps to subclasses. It allows subclasses to redefine certain steps of an algorithm without changing the algorithm’s structure.
- Can you explain the difference between a concrete class and an abstract class in OOP? When should each be used?
- In object-oriented programming (OOP), an abstract class is a class that cannot be instantiated and is meant to be a base class for one or more derived classes. An abstract class defines the interface for a group of related classes, but it does not provide a complete implementation for all of the methods it declares.
- On the other hand, a concrete class is a class that can be instantiated and provides a complete implementation for all of the methods it declares. A concrete class is a “normal” class that is not abstract and can be used to create objects.
- Here are some general guidelines for when to use an abstract class or a concrete class:
- Use an abstract class when you want to define the interface for a group of related classes, but you don’t want to provide a complete implementation for all of the methods. Abstract classes are useful for defining the “skeleton” of an algorithm while allowing subclasses to provide specific implementations for some of the steps.
- Use a concrete class when you want to provide a complete implementation for all of the methods in a class. Concrete classes are the “normal” classes that you use to create objects and define the behavior of those objects.
- Can you explain the difference between dynamic and static binding in OOP? When should each be used?
- In object-oriented programming (OOP), binding refers to the process of linking a method call to the method’s implementation. There are two types of binding: static binding and dynamic binding.
- Static binding, also known as early binding, occurs when the binding of a method call to its implementation is resolved at compile-time. This means that the method implementation is determined based on the type of the object at the time the code is compiled, rather than at runtime.
- Dynamic binding, also known as late binding, occurs when the binding of a method call to its implementation is not resolved until runtime. This means that the method implementation is determined based on the type of the object at the time the method is called, rather than at compile-time.
- Can you explain the difference between a final class and a sealed class in OOP? When should each be used?
- In object-oriented programming (OOP), a final class is a class that cannot be subclassed, while a sealed class is a class that cannot be subclassed or instantiated.
- A final class is a class that has been marked as “final” in the class definition, which means that it cannot be used as a base class for any other class. This is useful for preventing the class from being subclassed and changing the behavior of the class in unexpected ways.
- A sealed class is a class that has been marked as “sealed” in the class definition, which means that it cannot be used as a base class for any other class and cannot be instantiated directly. This is useful for preventing the class from being subclassed or instantiated, which can be helpful for enforcing encapsulation and maintaining the integrity of the class’s implementation.
- Can you explain the difference between a stack and a heap in OOP? When should each be used?
- In object-oriented programming (OOP), a stack and a heap are two different areas of memory that are used to store data.
- A stack is a region of memory that stores data in a last-in, first-out (LIFO) manner. It is used to store local variables and function parameters, as well as the return addresses of function calls. The stack is a fixed-size memory region that is managed by the operating system and is typically much smaller than the heap.
- A heap is a region of memory that is used to store dynamically-allocated variables. It is a much larger memory region than the stack and is not managed as strictly by the operating system. The heap is used to store objects that are created at runtime and is usually accessed through pointers.
- Here are some general guidelines for when to use a stack and a heap:
- Use a stack for storing local variables and function parameters, as well as the return addresses of function calls. The stack is a fixed-size memory region that is managed efficiently by the operating system and is suitable for storing small amounts of data that have a short lifetime.
- Use a heap for storing dynamically-allocated variables that may have a longer lifetime and may need to be accessed by multiple functions or threads. The heap is a larger memory region that is suitable for storing large amounts of data, but it is not as strictly managed by the operating system and may be more prone to memory leaks.
- Can you explain the difference between a synchronous and an asynchronous method in OOP? When should each be used?
- In object-oriented programming (OOP), a synchronous method is a method that executes in a blocking manner, while an asynchronous method is a method that executes in a non-blocking manner.
- A synchronous method is a method that is called and will not return until it has completed its execution. This means that the calling code is blocked until the method returns, which can make the program appear unresponsive if the method takes a long time to execute.
- An asynchronous method is a method that is called and returns immediately, without waiting for the method to complete its execution. This means that the calling code is not blocked and can continue to execute while the asynchronous method is running in the background.
- Here are some general guidelines for when to use a synchronous method and an asynchronous method:
- Use a synchronous method when you want to execute a task that has a relatively short execution time and does not need to interact with any external resources (such as a database or a network). Synchronous methods are simple and easy to understand, but they can make the program appear unresponsive if the task takes a long time to execute.
- Use an asynchronous method when you want to execute a task that may take a long time to execute or that needs to interact with external resources. Asynchronous methods allow the program to remain responsive while the task is being executed in the background, which can improve the user experience. However, asynchronous methods can be more complex to implement and may require the use of additional programming constructs (such as threads or promises).
Top OOP Interview Questions and Answers
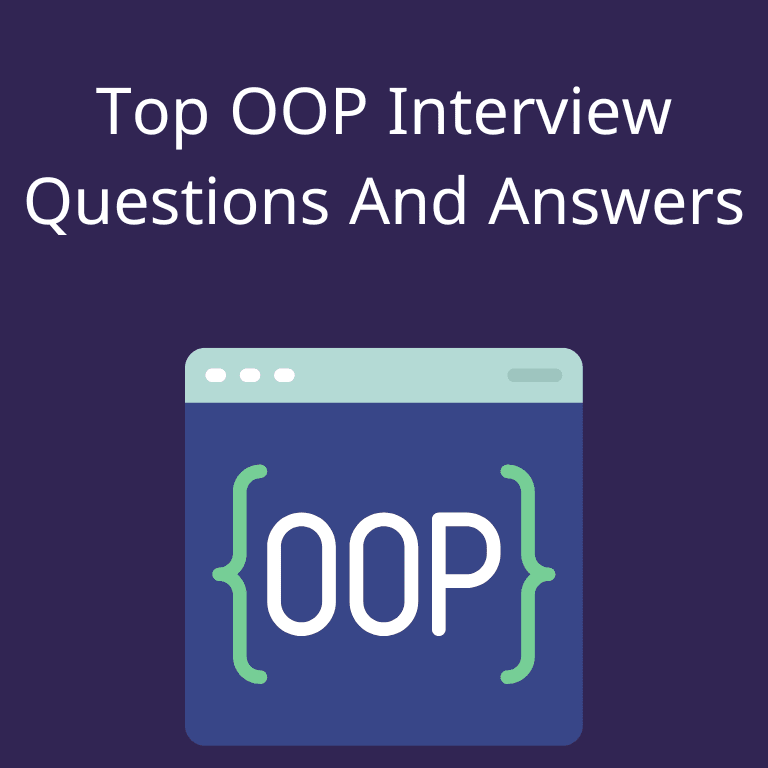